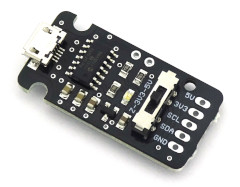
USB-I2C-Adapter board
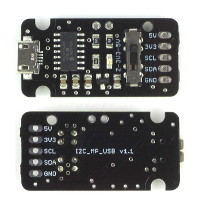
Buy on Fischl.de!
Buy on TinyTronics.nl!
Buy on Amazon.de!
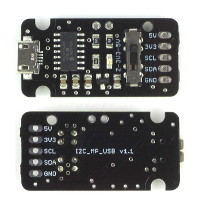
Buy on Fischl.de!
(€ 24,90 incl. 19% VAT excl. € 4 shipping)
Buy on TinyTronics.nl!
Buy on Amazon.de!
I2C-MP-USB - USB to I2C Interface
I2C-MP-USB is a interface to connect I²C devices via USB to a host system. It aims to easily control I2C slaves with highly portable code.- Open source Python library (Windows, MacOS, Linux)
- Native Linux support (compatible with kernel driver "i2c-tiny-usb")
- Wide signal voltage range (input high level: 2.1V - 5V)
- Integrated 4.7kOhm pullups switchable: 5V, 3.3V and off/Hi-Z
- Baudrate configuration via software (46k - 1M, default: 100k)
- Firmware sources available; bootloader for firmware updates
Contents
Software
A Python library is available for easy and rapid development on different operating systems. Alternatively a kernel driver can be used under Linux.Python library "pyI2C_MP_USB" (Windows, MacOS, Linux)
pyI2C_MP_USB is a Python library for the I2C-MP-USB adapter which allows easy, platform-independent access to I²C slaves.
The API is mostly identical to the SMBus package, porting of the control source code to other hardware is therefore possible without much effort.
Depending on the application, under Windows a libusb-1.0.dll is required. These can be found for various architectures on the libusb project homepage under "Download -> Windows Binaries". Please place the DLL in the application folder (e.g. in the "Scripts" folder for Anaconda Python).
Installation
The easiest way to install the library is using pip:pip install git+https://github.com/EmbedME/pyI2C_MP_USB
Drivers
The library uses libusb. On Linux and MacOS X no kernel driver is needed. Please use following tool for Windows to install the "WinUSB" driver: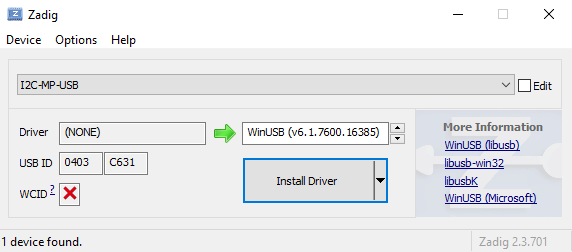
Depending on the application, under Windows a libusb-1.0.dll is required. These can be found for various architectures on the libusb project homepage under "Download -> Windows Binaries". Please place the DLL in the application folder (e.g. in the "Scripts" folder for Anaconda Python).
Example
Write one byte to an EEPROM 24C02 at address 0x10; then read it back:from i2c_mp_usb import I2C_MP_USB as SMBus import time bus = SMBus() bus.write_byte_data(0x50, 0x10, 0xA5) # Write byte to EEPROM time.sleep(0.1) # Give EEPROM time to flash data = bus.read_byte_data(0x50, 0x10) # Read byte from EEPROM print(data)
Throughput
A block read of 1000 bytes at 400kHz clock speed takes about 32ms on my test system (Ubuntu 16.04@ThinkPad X201). That means a transfer rate of 31.25 kBytes/s.Java library "jI2C_MP_USB" (Windows, MacOS, Linux)
jI2C_MP_USB is a Java library which provides access to I2C slaves with an I2C-MP-USB adapter.
It uses usb4java to talk over USB. Usb4java supports Linux (x86 32/64 bit, ARM 32/64 bit), OS X (x86 64 bit) and Windows (x86 32/64 bit).
Example
Read one byte from I²C device with address 0x50 from register address 0x10.I2C_MP_USB i2c = new I2C_MP_USB(); i2c.connect(); i2c.setBaudrate(100); byte test = i2c.readByteData(0x50, 0x10); System.out.println(String.format("%02X", test)); i2c.disconnect();
Kernel driver "i2c-tiny-usb" (Linux)
For Linux there is an alternative to the library: The driver "i2c-tiny-usb" is part of the official Linux kernel and is included in most distributions. When the adapter is plugged in, it is automatically detected and registered:
$ dmesg | tail usb 1-1.5.2.3: new full-speed USB device number 10 using ehci-pci usb 1-1.5.2.3: New USB device found, idVendor=0403, idProduct=c631 usb 1-1.5.2.3: New USB device strings: Mfr=1, Product=2, SerialNumber=3 usb 1-1.5.2.3: Product: I2C-MP-USB usb 1-1.5.2.3: Manufacturer: www.fischl.de usb 1-1.5.2.3: SerialNumber: 131E0000 i2c-tiny-usb 1-1.5.2.3:1.0: version 1.01 found at bus 001 address 010 i2c i2c-7: connected i2c-tiny-usb device usbcore: registered new interface driver i2c-tiny-usbExample using i2c-tools with an EEPROM 24C02 connected to the I2C-MP-USB.
- Detect devices on the I²C bus:
$ i2cdetect -y 7 0 1 2 3 4 5 6 7 8 9 a b c d e f 00: -- -- -- -- -- -- -- -- -- -- -- -- -- 10: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- 20: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- 30: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- 40: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- 50: 50 -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- 60: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- 70: -- -- -- -- -- -- -- --
- Write value 0x5A to EEPROM address 0x10:
$ i2cset -y 7 0x50 0x10 0x5A
- Read value from EEPROM address 0x10:
$ i2cget -y 7 0x50 0x10 0x5a
- Dump EEPROM content at address range 0x00-0x1F:
$ i2cdump -y -r 0x00-0x1F 7 0x50 b 0 1 2 3 4 5 6 7 8 9 a b c d e f 0123456789abcdef 00: ff ff ff ff ff ff ff ff ff ff ff ff ff ff ff ff ................ 10: 5a ff ff ff ff ff ff ff ff ff ff ff ff ff ff ff Z...............
- Load AT24 kernel module and register the EEPROM
$ modprobe at24 $ echo "24c02 0x50" > /sys/class/i2c-adapter/i2c-7/new_device
- Dump the eeprom content
$ hexdump -C /sys/bus/i2c/drivers/at24/7-0050/eeprom 00000000 ff ff ff ff ff ff ff ff ff ff ff ff ff ff ff ff |................| 00000010 5a ff ff ff ff ff ff ff ff ff ff ff ff ff ff ff |Z...............| 00000020 ff ff ff ff ff ff ff ff ff ff ff ff ff ff ff ff |................| * 00000100
- Unregister the EEPROM
$ echo "0x50" > /sys/class/i2c-adapter/i2c-7/delete_device
Firmware
Source code and HEX file
The firmware of I2C-MP-USB is available as precompiled HEX file and as C (XC8 compiler) source code.
Precompiled HEX and source code archive:
Precompiled HEX and source code archive:
i2c_mp_usb_firmware_v1.1.zip (2019-07-24) First public version
Firmware update via bootloader
Start the bootloader via software (e.g. start_bootloader.py) or via hardware jumper: short JP1 and plug in I2C-MP-USB - now the bootloader starts. Use a bootloader application (
MPHidFlash) to load the new firmware into I2C-MP-USB:

mphidflash -w i2c_mp_usb_firmware_v1.0.hex
Hardware
Schematic - I2C-MP-USB's circuit diagram
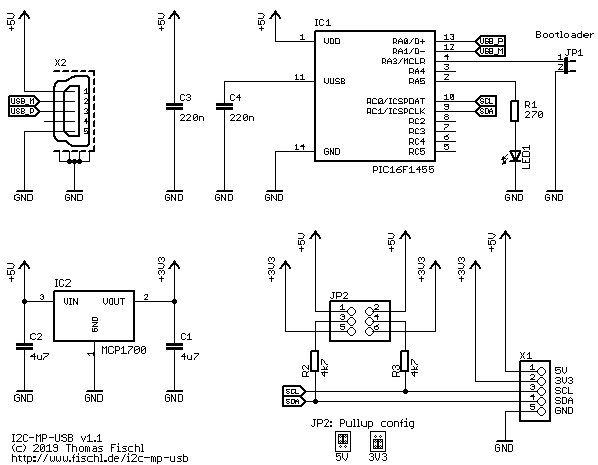
Partlist - Build your own I2C-MP-USB!
Here you find a list of all parts needed to build your own I2C-MP-USB board.
Partnumber | Value |
---|---|
C1, C2 | 4,7u |
C3, C4 | 220n |
IC1 | PIC16F1455 |
IC2 | MCP1700 |
LED1 | blue LED |
R1 | 270 |
R2, R3 | 4k7 |
X1 | 5pol 2.54mm |
X2 | USB-B |
Troubleshooting
WindowsError: [Error 126] The specified module could not be found
The libusb-DLL is missing. Please copy it into the python script folder. See: Drivers / libusb-1.0.dllLinux: "Permission denied" when accessing I2C devices

Linux: "LIBUSB_ERROR_BUSY" when using the Python library
If you use the Python library under Linux, deactivate the "i2c-tiny-usb" module, e.g. with$ rmmod i2c-tiny-usbOr blacklist it:
$ echo $'blacklist i2c-tiny-usb\n' > /etc/modprobe.d/i2c.conf
Linux: "LIBUSB_ERROR_ACCESS" as non-root
Add udev rule to give permissions to all users.sudo bash -c $'echo \'SUBSYSTEM=="usb", ATTRS{product}=="I2C-MP-USB", MODE="0666"\' > /etc/udev/rules.d/50-I2C_MP_USB.rules' sudo udevadm control --reload-rules
Gallery
Please tell me about your I2C-MP-USB project!![]() |
Read out the magnetic field strength from a SI7210. Post in the Mikrocontroller.net forum: I2C USB Interface with SI7210 (python script). by Rainer Wolf (09/2019) |
|
![]() |
Display text on a Grove - LCD RGB Backlight. Example Python script: grove_lcd_rgb.py by Thomas Fischl (10/2019) |
|
![]() |
Christof designed and built his own version of the I2C-MP-USB. by Christof Rueß, hobbyelektronik.org (03/2020) |
|
![]() |
Control MCP4017 (digital poti) with I2C-MP-USB under linux - with only three commands:
$ modprobe mcp4018 $ echo "mcp4017-103 0x2f" > /sys/class/i2c-adapter/i2c-8/new_device $ echo 12 > /sys/bus/i2c/drivers/mcp4018/8-002f/iio\:device0/out_resistance0_rawThe MCP4017-103 is a digital rheostat with 10 kOhm. The resistance can be set in 128 steps. The example above sets 12 (raw step value) * 78.740 (step resistance) + 75 (wiper resistance) = 1020 Ohm. by Thomas Fischl (09/2020) |
|
![]() |
Read smart battery data of notebook battery.
Example code on Github: smart_battery.py.
Tested with Li-Ion 84Wh pack for ThinkPad X201:
$python3 smart_battery.py Temperature (°C): 20.850000000000023 Voltage (V): 11.986 Current (mA): 0 RelativeStateOfCharge (%): 92by Thomas Fischl (10/2020) |
|
![]() |
Read Würth Elektronik WSEN-TIDS Temperature Sensor (Sensor: 2521020222501, Eval board: 2521020222591). Example python source code on Github: wsen_tids.py. Get and print temperature every 500ms: $ python3 wsen_tids.py Temperature in °C: 18.23 Temperature in °C: 18.26 Temperature in °C: 18.24 Temperature in °C: 18.22by Thomas Fischl (10/2020) |
|
![]() |
Enrico assembled the adapter on a breadboard to communicate with I2C slaves under Windows 10. He used Microchip Pickit 3 Programmer to program the microcontroller. by Enrico W. (10/2020) |
|
![]() |
Walter uses I2C-MP-USB to control the Digital-to-Analog converter MCP4725. Python code on Github: mcp4725_dac.py. by Walter S. (10/2020) |
|
![]() |
Read Würth Elektronik WSEN-HIDS Humidity Sensor with integrated Temperature Sensor (Sensor: 2525020210001, Eval board: 2525020210091). Example python source code on Github: wsen_hids.py. Get and print temperature and humidity every 500ms: $ python3 wsen_hids.py Temperature: 17.63 °C, Humidity: 59.89 % Temperature: 17.63 °C, Humidity: 59.91 % Temperature: 17.65 °C, Humidity: 59.82 %by Thomas Fischl (11/2020) |
|
![]() |
Control character LCD module (ERC802FS-1) with ST7032 controller. Example python source code on Github: lcd_st7032.py The printed circuit board is a custom designed board; the buttons are controlled by a dedicated microcontroller located on the back (not used in this example). by Thomas Fischl (11/2020) |
|
![]() |
Read Würth Elektronik WSEN-PADS Absolute Pressure Sensor (Sensor: 2511020213301, Eval board: 2511223013391). Example python source code on Github: wsen_pads.py. Get and print temperature and pressure every 500ms: $ python3 wsen_pads.py Temperature: 20.01 °C, Pressure: 98.041 kPa Temperature: 20.01 °C, Pressure: 98.042 kPa Temperature: 20.01 °C, Pressure: 98.042 kPaby Thomas Fischl (01/2021) |
|
![]() |
Read TI analog-digital converter ADS1015 (Breakout board: adafruit ADS1015). Example python source code on Github: ads1015.py. Get and print voltage every 500ms: $ python3 ads1015.py Voltage: 2.229 V Voltage: 2.229 Vby Thomas Fischl (06/2021) |
|
![]() |
Alexander realized an I2C hall sensor integration with low latency requirements in C/C++. He provides an example code for using the I2C-MP-USB in C/C++: i2c_mp_usb_cexample.c by Alexander Jasper (12/2023) |
Links
Other I²C interface projects/products



